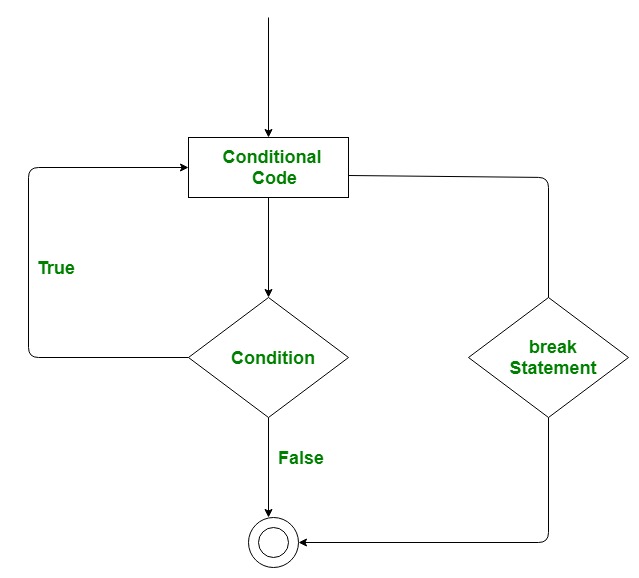
Python Break Program Execution
Forcing a stop is useful when your program locks up and won’t respond. The trick is to press Ctrl+C (the Ctrl key and the C key at the same time; don ‘ t press the Shift key). Make sure the Python window is active (by clicking the window) when you do — or you might close the wrong program!
Question
Is there any way in Python to stop program execution in the middle of the code?
Answer
Many programs written by scientists are not complex enough torequire the use of a debugger. Many of us prefer to check ourcode by putting in stop
statements and printingout the values of selected variables.
Ces cambridge engineering selector software s. The Cambridge Engineering Selector (CES) is a software package that has been produced to help answer these questions. Developed over a number of years by Mike Ashby and David Cebon, the Selector is now being marketed by their company, Granta Design. The selector makes use of an extensive database of information, and can also be linked to a company's own database for specific applications. CES Selector becomes GRANTA Selector with the upcoming 2020 release - a refreshed product, better than ever in support of your key materials decisions. Materials selection. GRANTA Selector is the standard tool for materials selection. Innovate, evolve products, solve materials problems, confirm and validate your choices.
Python does not have a stop
statement.I looked really really hard for one. There is a break
statement for getting out of loops, and there's an elegantexception handler, but nothing exists in the language to just stop program execution to let you inspect the state of theprogram.
Thankfully, through one of the methods in the Pdbdebugger (included with the standard Python distribution),we can simulate a stop
statement. Ifwe are executing code in an interpreter window, the programwill even stop execution and allow us to inspect any variablewe want, like in IDL.
I learned how to do this from Stephen Berg's excellent articleDebugging in Python. Here I'll summarize how touse Pdb
to stop program execution.
Say we have the following simple program:
a = 1
b = a+2
print 'Line 3'
print 'Line 4'
and we wish to put a 'stop' after b = a+2
,like this:
The description of Fake Driving Licence India. Fake ID Card Maker or Fake ID Generator For Driving License is your answer to pranking your friends into believing that you already have a Driving License. If you have ever felt the need to have a fake ID then Fake Driving License Maker or Fake ID Generator is your wish fulfilled. There are also some who have a driving permit but they have lost it. Making a driving licence takes a bit of time. The fake driving licence generator can help you kill that time while your license is made. This is because it is important to have a driving licence card, Just imagine that you are driving. If a cop pulls you over and asks for a licence.
a = 1
stop
b = a+2print 'Line 3'
print 'Line 4'
To do this, I add the following snippet of code at the 'stop':
import pdb #@@@
pdb.set_trace() #@@@
print 'stop here' #@@@
The @@@
comments are there so I can easily find thoselines to remove them after I've finished debugging.So, the program now reads:
a = 1
b = a+2
import pdb #@@@
pdb.set_trace() #@@@
print 'stop here' #@@@
print 'Line 3'
print 'Line 4'
When you run the program, after b = a+2
executes, you will be put into the Pdb debugger. YourPython session (I'm assuming you're using the interactiveinterpreter) will look something like this:
>>> execfile('foo.py')
--Return--
> /usr/lib/python2.1/pdb.py(895)set_trace()->None
-> Pdb().set_trace()
(Pdb)
Pdb has this interesting feature in that when you first invoke it(via the pdb.set_trace()
call), it enters the 'Pdb'mode but doesn't do or know anything. For instance, if you tryto inspect a program variable, it doesn't know the variableexists.
To see everything in your program, you have to step forward oneline, by typing n
at the (Pdb)
prompt.You'll now get something like:
(Pdb) n
> /home/jlin/foo.py(5)?()
-> print 'stop here' #@@@
(Pdb)

At this point, you can print whatever is currently defined inyour program. In fact, you can now execute any interactivePython statement (except definitions like loops, etc.)that you want at the (Pdb)
prompt. There aresome exceptions; sometimes, you have to add an exclamation point(!) to the statement in order for it to work. SeeDebugging in Python for details.
So, if you're interested in seeing the value of b
,at the (Pdb)
prompt type:
(Pdb) !b
3
(Pdb)
and the value of b
is returned. Note that b
without the !
means 'set breakpoint' in Pdb. We add the!
so Pdb knows to return the value of variable b
,not to set a breakpoint.
When you're done, press q
to get out ofPdb. You'll get a bunch of traceback messages, before youreturn to the interactive Python prompt (>>>
), but just ignore those messages.
Is this hard?
I am introducing Python to my students and want to make a simple, interactive program that will, while it's running, 'listen' for any keyboard events. That is, I don't want to include an 'input' statement - that would pause execution till someone answered. I just want it to keep running, say, printing an 'a' on the screen every 10 seconds, until the user types a 'b'. Then printing a 'b' every 10 seconds till the user types in some other character..
It seems that this should not be hard; something with sys.stdin, perhaps. The hours I've spent on it are getting embarrassing.
I've fooled around with it and looked though books and the only solution I've come up with was to import pygame.py and use its event handling. This seems like massive overkill for what should be an easy matter.
I'm using IDLE with MacPython 2.5.1 on Mac OS 10.4.11
Thanks for any help on this problem.
Neal C.
- 5 Contributors
- forum 6 Replies
- 167 Views
- 1 Month Discussion Span
- commentLatest Postby Freaky_ChrisLatest Post